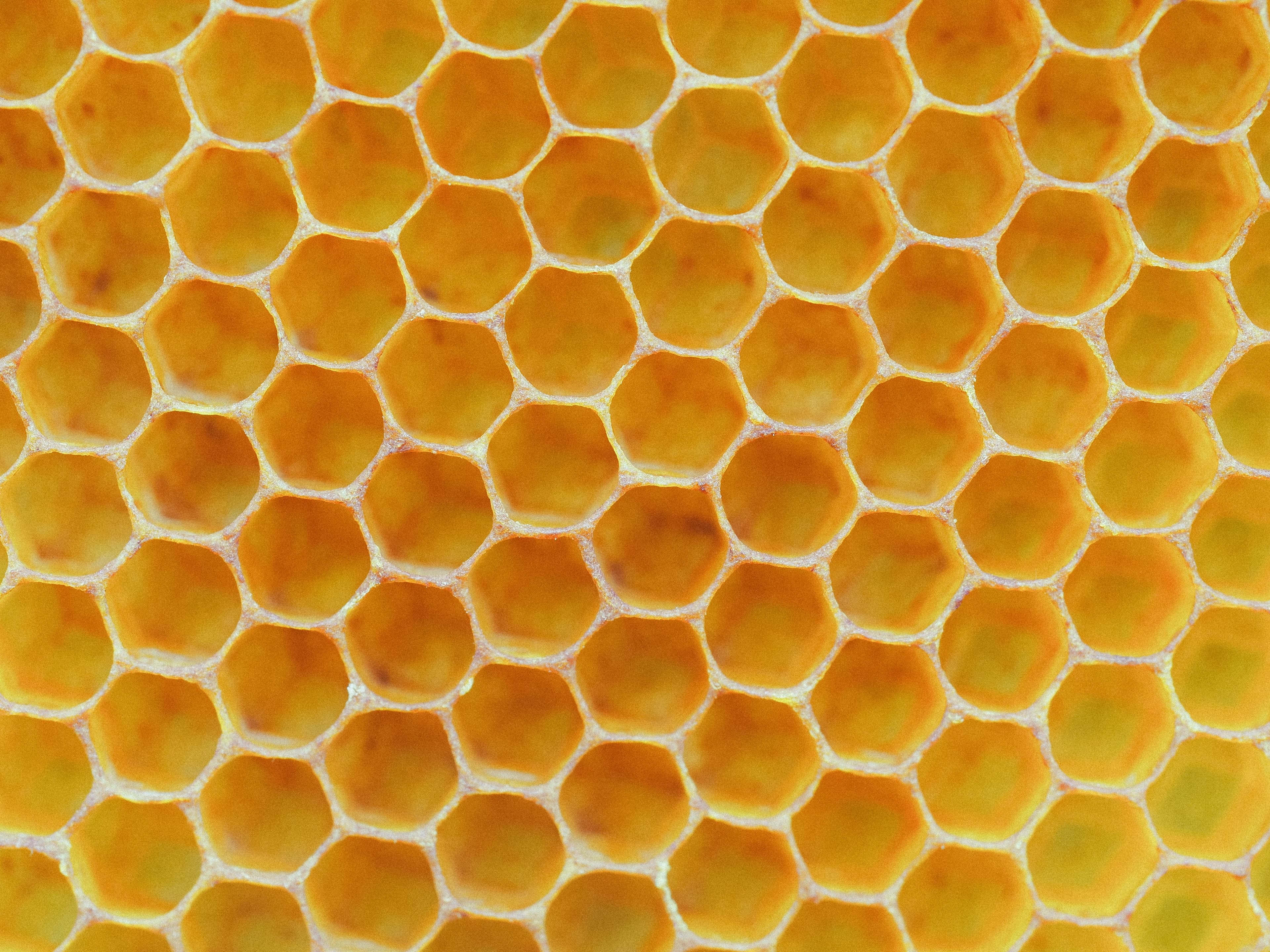
Setting up GraphQL in Next.js
Nov 24th 2022 . Moe Rabay'a
Introduction
In this article we will quickly setup our NextJS application with GraphQL (Apollo Server). If you not familiar with NextJS, I highly recommend you to read and get started with NextJS.
Setup
If you haven't setup your NextJS application yet, go ahead and create your application.
npx create-next-app mountain-view
Once you are done with this, we will begin adding our graphql dependencies. We will need to install @apollo/server
, graphql
and @as-integrations/next
, which allow us to run an Apollo server in our application:
npm install @apollo/server graphql @as-integrations/next
Structure
If you are familiar with graphql, we know that we have two main components in any
graphql application. That would be resolvers
and schema
. Also we need to create
our graphql end point, this would also allow us to access our playground.
├── apollo
| ├── resolvers
| | └── index.js
| └── schemas
| └── index.js
└── pages
├── api
| └── graphql.js
└── index.js
Let's create a simple query that has a simple method that returns all posts. In api/schemas/index.js
export const typeDefs = `#graphql
type Post {
id: ID
name: String
description: String
content: String
}
type Query {
getPosts: [Post]
}
`;
We need to create resolvers for our new two methods, we can use github api to return
these posts In api/resolvers/index.js
export const resolvers = {
Query: {
getPosts: async () => {
try {
const posts = [
{
id: "random-id-7322371",
name: "GraphQL Post",
description: "This is a blog post.",
},
];
return posts;
} catch (error) {
throw error;
}
},
},
};
and that's pretty much all we need, now if you start your NextJS application and go to /api/graphql you will see this.
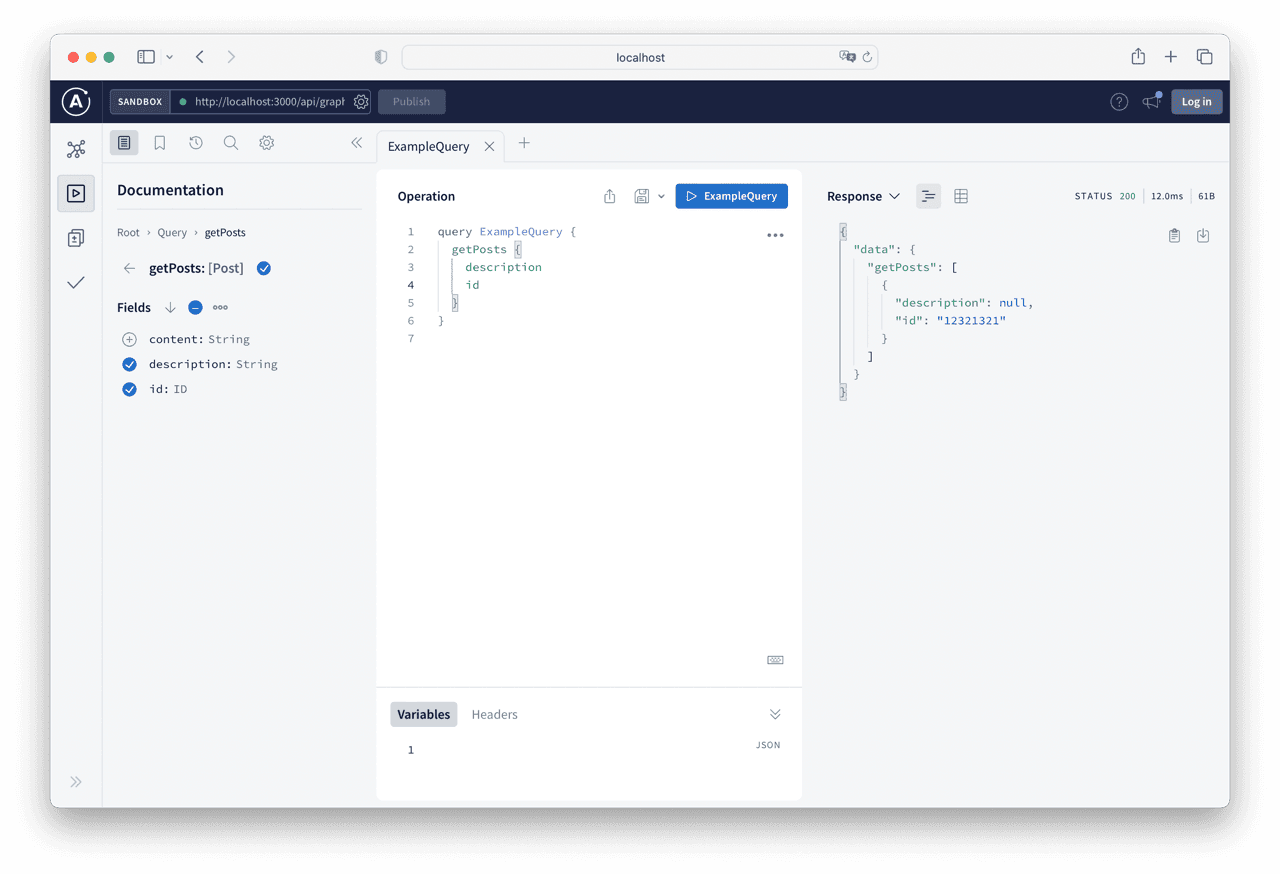
Happy coding!